Android Shared Element Transitions- Catchy Enough?
- Prakhar Srivastava
- Aug 31, 2020
- 2 min read
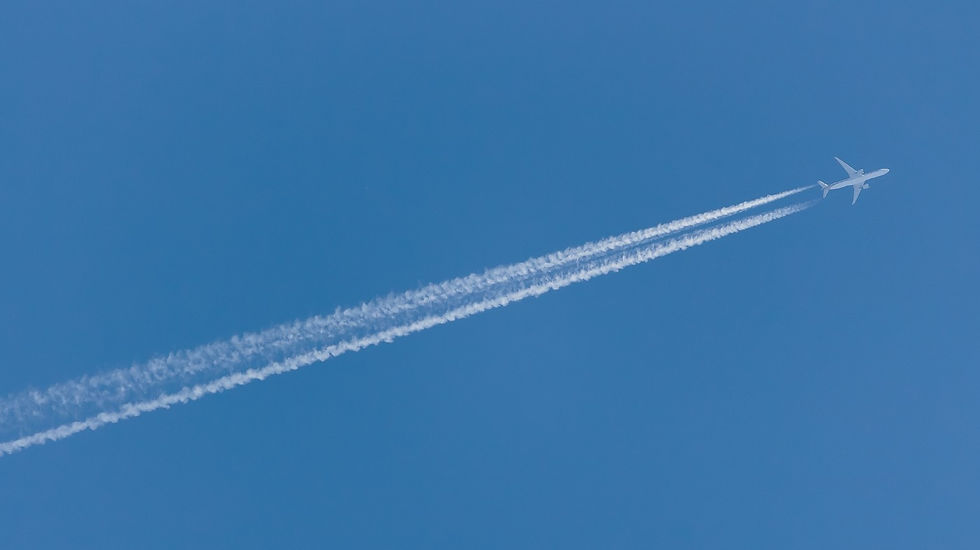
We are going to do a simple android shared element transition today. The shared element transition is one of the designs that any user loves to engage with.
For those who are new to the concept of shared element transition, we are going to do this today:
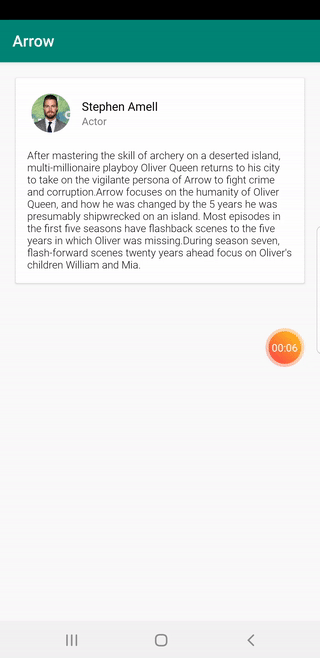
Before we start, here is the full source code: https://github.com/shree-vastava/SharedElementTransition
To achieve this animation, we need only 2 simple steps:
Define same transition name for each element in both the xml.
Define transition in the intent to next activity
Transition Name in XML
In this step, we will define the transition name to the elements we want to animate in the xml, in our case the circular image, name and occupation.
Note that the image, name and occupation are present in both activities. The transition name for one element should be same in both the activities. For example, if we are giving the transition name “imageTrans” to the image in activity 1, we shall give the same name to the image in activity 2.
So here is the code of image, name and occupation from the activity_main.xml (activity1):
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/personImage"
android:layout_width="50dp"
android:layout_height="50dp"
android:scaleType="centerCrop"
android:transitionName="picture"<TextView
android:id="@+id/personName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Stephen Amell"
android:textColor="#000"
android:transitionName="name"
android:fontFamily="@font/roboto_regular"
android:textSize="14sp"/>
<TextView
android:id="@+id/personName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Stephen Amell"
android:textColor="#000"
android:transitionName="name"
android:fontFamily="@font/roboto_regular"
android:textSize="14sp"/>
<TextView
android:id="@+id/personOccupation"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Actor"
android:transitionName="occupation"
android:fontFamily="@font/roboto_regular"
android:textSize="12sp"/>
Here the transition names for the elements are following personImage: picture personName: name personOccupation: occupation
Now the elements from the activity_details.xml (activity2):
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="match_parent"
android:layout_height="120dp"
android:scaleType="centerCrop"
android:layout_marginTop="20dp"
android:transitionName="picture"
android:src="@drawable/stephen"/>
<TextView
android:id="@+id/personName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20sp"
android:layout_marginTop="10dp"
android:transitionName="name"
android:textColor="#B84D30"
android:layout_gravity="center"
android:fontFamily="@font/roboto_regular"
android:text="Stephen Amell"/>
<TextView
android:id="@+id/personOccupation"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20sp"
android:textColor="#5565AF"
android:transitionName="occupation"
android:layout_gravity="center"
android:fontFamily="@font/roboto_light"
android:text="Actor"/>
Note that the transition names are same for the respective elements.
Implement transition in Kotlin
Let’s see what is required in the kotlin/java class to make it work. Here we won’t use the intent with only one argument. So, we will use:
startActivity(intent, bundle)
So the complete code comes out to be:
profile_layout.setOnClickListener {
var intent = Intent(this,DetailsActivity::class.java)
startActivity(intent, ActivityOptions.makeSceneTransitionAnimation(this,
android.util.Pair(personImage,personImage.transitionName),
android.util.Pair(personName,personName.transitionName),
android.util.Pair(personOccupation,personOccupation.transitionName)).toBundle())
}
There is no code required in the details activity.
Cheers!!
Here is the full source code:
Comments